KDevelop5/Manual/Debugging programs
Debugging programs in KDevelop
Running a program in the debugger
Once you have a launch configured (see Running programs), you can also run it in a debugger: Select the menu item , or hit F9. If you are familiar with gdb, the effect is the same as starting gdb with the executable specified in the launch configuration and then saying Run
. This means that if the program calls abort()
somewhere (e.g. when you run onto a failing assertion) or if there is a segmentation fault, then the debugger will stop. On the other hand, if the program runs to the end (with or without doing the right thing) then the debugger will not stop by itself before the program is finished. In the latter case, you will want to set a breakpoint on all those lines of your code base where you want the debugger to stop before you run the debug launch. You can do that by moving the cursor on such a line and selecting the menu item , or right-clicking on a line and selecting from the context menu.
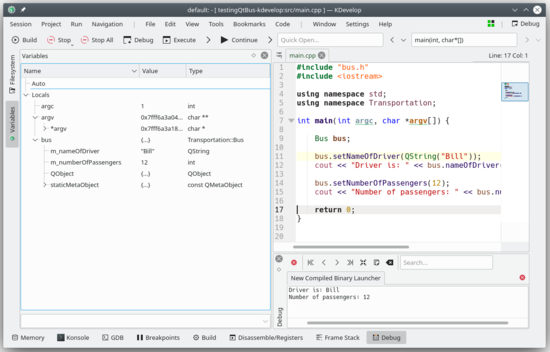
Running a program in the debugger will put KDevelop in a different mode: it will replace all the "Tool" buttons on the perimeter of the main window by ones that are appropriate for debugging, rather than for editing. You can see which of the modes you are in by looking at the top right of the window: there is a button named Tools and views.
in editing mode, and a button named in debugging mode; clicking on them allows you to switch back and forth between the two modes; each mode has a set of tool views of its own, which you can configure in the same way as we configured the tools in the sectionOnce the debugger stops (at a breakpoint, or a point where abort()
is called) you can inspect a variety of information about your program. For example, in the image above, we have selected to show the programs output. The tool at the bottom (roughly equivalent to gdb's "backtrace" and "info threads" commands) that shows the various threads that are currently running in your program at the left, and how execution got to the current stopping point at the right (here: main()
called Bus bus;
; the list would be longer had we stopped in a function called by bus
itself). On the left, we can inspect local variables including the current object (the object pointed to by the this
variable).
From here, there are various possibilities you can do: You can execute the current line (F10, gdb's "next" command), step into the functions (F11, gdb's "step" command), or run to the end of the function (F12, gdb's "finish" command). At every stage, KDevelop updates the variables shown at the left to their current values. You can also hover the mouse over a symbol in your code, e.g. a variable; KDevelop will then show the current value of that symbol and offer to stop the program during execution the next time this variable's value changes. If you know gdb, you can also click on the tool button at the bottom and have the possibility to enter gdb commands, for example in order to change the value of a variable (for which there doesn't currently seem to be another way).
Attaching the debugger to a running process
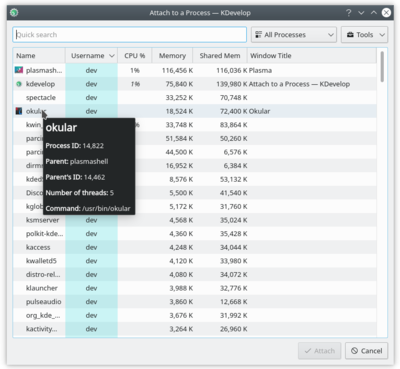
Sometimes, one wants to debug a program that's already running. One scenario for this is debugging parallel programs using MPI, or for debugging a long running background process. To do this, go to the menu entry , which will open a window like the one above. Select the program you wish to attach to and then click . If you fail to attach to the process and get the following result:
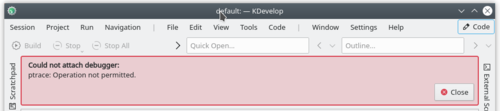
Attaching a debugger to a running program is not allowed by default on most Linux distributions. To do so, you first need to know a little about PTRACE system that is used for debugging. Generally, the default setting is a scope of "1", which limits PTRACE to direct child processes only (e.g. "gdb name-of-program" and "strace -f name-of-program" work, but gdb's "attach" and "strace -fp $PID" do not). A PTRACE scope of "0" is the more permissive mode.
There are two ways to change this on Ubuntu, if you are running a different distribution, your requirements may be different. On Ubuntu, one way is to edit the /etc/sysctl.d/10-ptrace.conf file and change kernel.yama.ptrace_scope to 0. After editing the file run the following command:
sudo service procps restart
You should now be able to attach to a running process.
The other way that is the more secure way is to run:
echo 0 | sudo tee /proc/sys/kernel/yama/ptrace_scope
when you want to use the feature, and:
echo 1 | sudo tee /proc/sys/kernel/yama/ptrace_scope
when you have completed your need for attaching to a running process.
After setting your ptrace_scope to 0, you will want to select the program that matches your currently open project in KDevelop.
This list of programs can be confusing because it is often long as in the case shown here. You can make your life a bit easier by going to the dropdown box at the top right of the window. The default value is
, i.e. all programs that are run by any of the users currently logged into this machine (if this is your desktop or laptop, you're probably the only such user, apart from root and various service accounts); the list doesn't include processes run by the root user, however. You can limit the list by either choosing , removing all the programs run by other users. Or better still: Select , which removes a lot of processes that are formally running under your name but that you don't usually interact with, such as the window manager, background tasks and so on that are unlikely candidates for debugging.Once you have selected a process, attaching to it will get you into KDevelop's debug mode, open all the usual debugger tool views and stop the program at the position where it happened to be when you attached to it. You may then want to set breakpoints, viewpoints, or whatever else is necessary and continue program execution by going to the menu item
.Some useful keyboard shortcuts
Debugging | |
---|---|
F10 | Step over (gdb's "next") |
F11 | Step into (gdb's "step") |
F12 | Step out of (gdb's "finish") |